Here’s a step-by-step tutorial on how to create a countdown timer using HTML, CSS, Bootstrap, and JavaScript. This tutorial allows the user to input the desired countdown time.
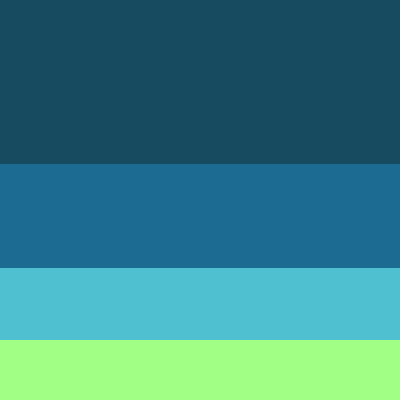
Step 1: Set up the HTML structure
Start by creating an HTML file and add the necessary HTML structure. Include the Bootstrap CSS and JavaScript files for styling and functionality.
<!DOCTYPE html>
<html>
<head>
<title>Countdown Timer Tutorial</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<h1>Countdown Timer</h1>
<div class="form-group">
<label for="time-input">Enter time in seconds:</label>
<input type="number" class="form-control" id="time-input" placeholder="Enter time">
<small class="text-muted">Please enter a positive integer value.</small>
</div>
<button class="btn btn-primary" onclick="startCountdown()">Start Countdown</button>
<div id="timer" class="mt-3"></div>
</div>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: Create the CSS styles
Add some CSS styles to make the countdown timer visually appealing. Create a separate CSS file called styles.css
and include it in the HTML file.
#timer {
font-size: 48px;
text-align: center;
margin-top: 20px;
}
Step 3: Implement the JavaScript logic
Create a JavaScript file called script.js
and link it to the HTML file. This is where we’ll write the countdown timer logic.
function startCountdown() {
var timeInput = document.getElementById('time-input');
var timer = document.getElementById('timer');
var timeInSeconds = parseInt(timeInput.value);
if (isNaN(timeInSeconds) || timeInSeconds <= 0) {
alert('Please enter a valid positive integer.');
return;
}
var countdownInterval = setInterval(function() {
if (timeInSeconds <= 0) {
clearInterval(countdownInterval);
timer.innerHTML = 'Countdown finished!';
} else {
timer.innerHTML = formatTime(timeInSeconds);
timeInSeconds--;
}
}, 1000);
}
function formatTime(timeInSeconds) {
var minutes = Math.floor(timeInSeconds / 60);
var seconds = timeInSeconds % 60;
return minutes.toString().padStart(2, '0') + ':' + seconds.toString().padStart(2, '0');
}
Step 4: Test the countdown timer
Save all the files in the same directory and open the HTML file in a web browser. You should see a countdown timer input field, a “Start Countdown” button, and a timer display.
Enter the desired countdown time in seconds and click the “Start Countdown” button. The timer will start ticking down, updating every second until it reaches zero. At that point, the message “Countdown finished!” will be displayed.
Congratulations 🥳 ! You have created a countdown timer with user-inputted time using HTML, CSS, Bootstrap, and JavaScript.